sketch
//maddy cha
//section e
//mecha@andrew.cmu.edu
//project-10
var clouds = [];
var birdStrings = [];
var birdImgs = [];
var birds = [];
var birdTalk = ["hello", "hey", "hi", "howdy", "help me", "i love bread", "thank you", "good bye", "hm"];
//preloads all illustrator bird files locally
function preload() {
birdStrings[0] = "https://i.imgur.com/LMwUiTl.png";
birdStrings[1] = "https://i.imgur.com/2DClEHo.png";
birdStrings[2] = "https://i.imgur.com/LoaUSI2.png";
birdStrings[3] = "https://i.imgur.com/yMJKBYw.png";
birdStrings[4] = "https://i.imgur.com/jF6usAy.png";
birdStrings[5] = "https://i.imgur.com/GPZ41QC.png";
birdStrings[6] = "https://i.imgur.com/15jvGyQ.png";
birdStrings[7] = "https://i.imgur.com/XcxgSGA.png";
for (var b = 0; b < 8; b++) {
birdImgs[b] = loadImage(birdStrings[b]);
}
}
//creates canvas initialized with birds, clouds
function setup() {
createCanvas(480, 360);
//initializes three birds at set x location
birds[0] = birdPicture(50);
birds[1] = birdPicture(200);
birds[2] = birdPicture(400);
clouds[0] = cloudPicture(60);
clouds[1] = cloudPicture(290);
}
function draw() {
//sets background to be dark blue of bird
background(81,81,122);
//calls function that draws mountains
mountains();
//draws clouds behind first mountain
updateClouds();
addNewClouds();
mountains2();
//draws horizon line
noStroke();
fill(250, 240, 240);
rect(0, 290, width, height);
//draws birds
updateBirds();
addNewBirds();
}
function updateClouds(){
//change the x location of each cloud
for(var i = 0; i < clouds.length; i++){
clouds[i].cmove();
clouds[i].cdraw();
}
}
function addNewClouds(){
//adds new clouds with same probability of a number drawn between 0 and 1.2 being less than 0.003
var probability = 0.003;
if(random(0,1.2) < probability) {
clouds.push(cloudPicture(width));
}
}
function cloudPicture(cloudX){
var cloudPicture = {
cx: cloudX,
cy: random(40,140),
velo: 0.4,
cmove: cloudMove,
cdraw: drawCloud,
cwidth: random(60,130),
cheight: random(20,30)
}
return cloudPicture;
}
function cloudMove() {
this.cx -= this.velo;
}
function drawCloud(){
noStroke();
fill(255);
rect(this.cx, this.cy, this.cwidth, this.cheight,30);
rect(this.cx + this.cwidth-(this.cwidth/1.2), this.cy-this.cheight+(this.cheight/2), this.cwidth/2, this.cheight,30);
}
function updateBirds() {
//change the x location of each of the birds
for (var i = 0; i < birds.length; i++) {
birds[i].bmove();
birds[i].bdraw();
}
}
function addNewBirds() {
//adds new birds with same probability of a number drawn between 0 and 1.2 being less than 0.004
var probability = 0.004;
if (random(0, 1.2) < probability) {
birds.push(birdPicture(width));
}
}
function birdPicture(startX) {
var birdPicture = {
x: startX,
vel: 1,
type: floor(random(0, 7)),
bmove: birdMove,
bdraw: drawBird,
speak: bTalk(),
talkProbability: tP()
}
return birdPicture;
}
//coin toss equivalent
function tP() {
var talkProb = false;
if (random(0, 1) > 0.8) {
return true;
}
return talkProb;
}
//adds velocity to x to make birds scroll horizontally
function birdMove() {
this.x -= this.vel;
}
function drawBird() {
//if bird is shorter, draw lower on page
if (this.type == 0 || this.type == 1 || this.type == 4 || this.type == 5) {
image(birdImgs[this.type], this.x, 213);
//will draw text for this bird depending on probability
if (this.talkProbability == true) {
fill(255);
text(this.speak, this.x + 20, 193)
}
}
//if bird is taller, draw higher on page
else {
image(birdImgs[this.type], this.x - 30, 180);
//will draw text for this bird depending on probability
if (this.talkProbability == true) {
fill(255);
text(this.speak, this.x, 160);
}
}
}
//returns strings in birdTalk array
function bTalk() {
return birdTalk[int(random(birdTalk.length))];
}
function mountains() {
var terrainSpeed = 0.0002;
var terrainDetail = 0.005;
push();
beginShape();
noStroke();
fill(190, 160, 180);
vertex(0, height);
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 1, 0, height);
vertex(x, y - 90);
}
vertex(width, height);
endShape();
pop();
}
function mountains2() {
var terrainSpeed = 0.0004;
var terrainDetail = 0.003;
push();
beginShape();
noStroke();
fill(140,120,150);
vertex(0, height);
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 1, 0, height);
vertex(x, y-40);
}
vertex(width, height);
endShape();
pop();
}
For this project, I decided to do a landscape with birds, as I had done a lot of projects with birds previously.
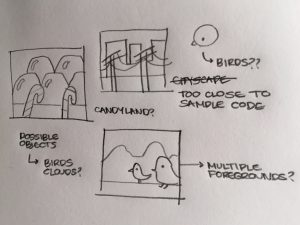
Rather than create the birds through coding, I decided to use illustrator in order to create vectors that I could implement in the code. In order to do this, I ran a preload function that populated an array with my images uploaded to imgur.


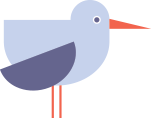
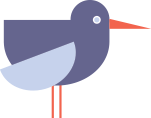
I created two objects–birds and clouds–and populated arrays with them in order to have them appear in my environment.