sketch
var t1;
var t2;
var t3;
var t4;
var t5;
var t6;
function setup() {
createCanvas(480,480);
background(0);
t1 = makeTurtle(0, 1*height/6);
t2 = makeTurtle(0, height/2);
t3 = makeTurtle(0, 5*height/6);
t4 = makeTurtle(0,height);
t5 = makeTurtle(0,0);
t6 = makeTurtle(width, 1*height/2);
t7 = makeTurtle(0, 2*height/6);
t8 = makeTurtle(0, 4*height/6);
}
function draw() {
followingDraw();
}
//the fucntion below creates turtles that constantly turn towards the mouse, creating a snake-like effect
function followingDraw (){
t1.penDown();
t1.setColor(255);
t1.forward(2);
t1.turnToward(mouseX, mouseY, 10);
t2.penDown();
t2.setColor(255);
t2.forward(2);
t2.turnToward(mouseX, mouseY, 10);
t3.penDown();
t3.setColor(255);
t3.forward(2);
t3.turnToward(mouseX, mouseY, 10);
t4.penDown();
t4.setColor(255);
t4.forward(2);
t4.turnToward(mouseX, mouseY, 10);
t5.penDown();
t5.setColor(255);
t5.forward(2);
t5.turnToward(mouseX, mouseY, 10);
t7.penDown();
t7.setColor(255);
t7.forward(2);
t7.turnToward(mouseX, mouseY, 10);
t8.penDown();
t8.setColor(255);
t8.forward(2);
t8.turnToward(mouseX, mouseY, 10);
//this is the lone red line from the right
var red = color(255,0,0);
t6.penDown();
t6.setColor(red);
t6.forward(2);
t6.turnToward(mouseX, mouseY, 10);
}
function turtleLeft(d) {
this.angle -= d;
}
function turtleRight(d) {
this.angle += d;
}
function turtleForward(p) {
var rad = radians(this.angle);
var newx = this.x + cos(rad) * p;
var newy = this.y + sin(rad) * p;
this.goto(newx, newy);
}
function turtleBack(p) {
this.forward(-p);
}
function turtlePenDown() {
this.penIsDown = true;
}
function turtlePenUp() {
this.penIsDown = false;
}
function turtleGoTo(x, y) {
if (this.penIsDown) {
stroke(this.color);
strokeWeight(this.weight);
line(this.x, this.y, x, y);
}
this.x = x;
this.y = y;
}
function turtleDistTo(x, y) {
return sqrt(sq(this.x - x) + sq(this.y - y));
}
function turtleAngleTo(x, y) {
var absAngle = degrees(atan2(y - this.y, x - this.x));
var angle = ((absAngle - this.angle) + 360) % 360.0;
return angle;
}
function turtleTurnToward(x, y, d) {
var angle = this.angleTo(x, y);
if (angle < 180) {
this.angle += d;
} else {
this.angle -= d;
}
}
function turtleSetColor(c) {
this.color = c;
}
function turtleSetWeight(w) {
this.weight = w;
}
function turtleFace(angle) {
this.angle = angle;
}
function makeTurtle(tx, ty) {
var turtle = {x: tx, y: ty,
angle: 0.0,
penIsDown: true,
color: color(128),
weight: 1,
left: turtleLeft, right: turtleRight,
forward: turtleForward, back: turtleBack,
penDown: turtlePenDown, penUp: turtlePenUp,
goto: turtleGoTo, angleto: turtleAngleTo,
turnToward: turtleTurnToward,
distanceTo: turtleDistTo, angleTo: turtleAngleTo,
setColor: turtleSetColor, setWeight: turtleSetWeight,
face: turtleFace};
return turtle;
}
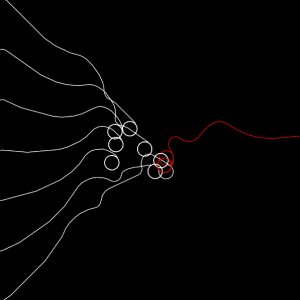
I thought that this project was quite simple, yet a created a very alluring graphic. I honestly just went for this project without any sketches, only knowing that I wanted to create lines that either followed by mouse or ran away from it. However, after reaching this point of the project I didn’t really see a point in adding more, because I thought that would ruin the effect of the simplicity of the project. Finally, although it wasn’t intentional I thought that the circles that are often formed by quickly moving the mouse back and forth created a notion that the “piece” generated by the program was finished, thus becoming an “artwork generator”.