sketch
var underlyingImage; //global variable for image that is not shown
function preload() {
var myImageURL = "https://i.imgur.com/wSnQo5j.jpg"; //image of dad
underlyingImage = loadImage(myImageURL);
}
function setup() {
createCanvas(700, 600);
background(0); //black background
underlyingImage.loadPixels(); //load pixels
frameRate(10); //slow placement of objects when pixels called
}
function draw() {
var px = random(width); //random x and y variables to place something at
var py = random(height);
var ix = constrain(floor(px), 0, width-1); //constrain the x and y variables for color sake
var iy = constrain(floor(py), 0, height-1);
var theColorAtLocationXY = underlyingImage.get(ix, iy); //getting color of each pixel
var r = theColorAtLocationXY[0]; //r value of color
var g = theColorAtLocationXY[1]; // g value of pixel color
var b = theColorAtLocationXY[2]; //b value of color
var r2 = 255- r; //opposite r value
var g2 = 255-r; //opposite g value
var b2 = 255 - r; //opposite b value
noStroke();
if(g < 150 & b < 150) { //if g and b values are lower, make a clear ellipse of that pixel color and a smaller circle inside that is slightly redder
fill(r, g, b, 170);
ellipse(px, py, 20, 20);
fill(r + 10, g - 10, b - 10);
ellipse(px, py, 5, 5);
}
if(b > 230) { //if the b value is large, make an ellipse that is bluer than the pixel and make an arrangement of other blue circles surrounding it
fill(r- 10, g - 10, b + 10, 150);
ellipse(px, py, 5, 5);
var numObjects = 5;
var angleObject = 360/numObjects;
for(var i = 0; i < numObjects; i++) {
var posX = px + random(2, 7) *cos(radians(angleObject*i) );
var posY = py + 5 *sin(radians(angleObject*i) );
ellipse(posX, posY, 10, 10);
}
}
if(g > 150) { //if the green value is low, make a circle slightly greener that is random in radius
fill(r, g + 10, b, 100);
var pointSize = random(10, 60);
ellipse(px, py, pointSize, pointSize);
}
if(r > 200) { //if the r value is large, make an arrangement of circles with strokes that is slightly redder
var numObjects = 8;
var angleObject = 360/numObjects;
for(var i = 0; i < numObjects; i++) {
var posX = px + random(-5, 5) *cos(radians(angleObject*i) );
var posY = py + 5 *sin(radians(angleObject*i) );
noFill();
stroke(r +20, g - 10, b - 10);
strokeWeight(random(.25, 5));
ellipse(posX, posY, 10, 10);
}
}
if(r < 50 & g < 50 && b < 50) { //if all r g b values are on the lower end, make a row of five circles of that color which is moving downwards to the right
for(var i = 0; i < 5; i++) {
fill(theColorAtLocationXY, 300 - i*40);
ellipse((px - 15) +5* i, (py-15)+ 5* i, 2, 2);
}
}
else {
noFill(); //if the pixel does not meet any of the conditional statements, make a blank circle with the strokeWeight random and the stroke of the color at the pixel
stroke(theColorAtLocationXY);
strokeWeight(random(1, 8));
ellipse(px,py, 10, 10);
}
var sx = mouseX; //when the mouse runs over the canvas
var sy = mouseY;
var mx = constrain(floor(sx), 0, width-1); //constrain the mouse value for color
var my = constrain(floor(sy), 0, height-1);
var theColorAtLocationMxMy = underlyingImage.get(mx, my); //color at point of mouse
var drawSize = dist(pmouseX, pmouseY, mouseX, mouseY); //distance between mouse coordinates at previous to current point
fill(r2, g2, b2, 200); //opposite color
ellipse(sx, sy, drawSize/10, drawSize/10); //draw a circle with mouse stroke depending on the mouse speed
if(mouseIsPressed) { //if the mouse is pressed, make a circle the color of that pixel
fill(theColorAtLocationMxMy);
ellipse(mouseX, mouseY, 7, 7);
}
}
I liked playing with opacity in the shapes we make in class. I think the randomization of pixels along with the overlapping of the colors is nice.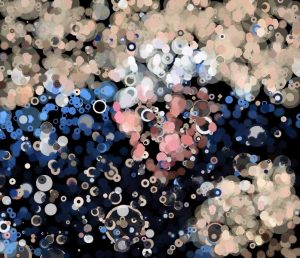
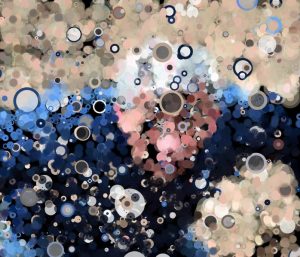
