//Cora Hickoff
//Section D
//chickoff@andrew.cmu.edu
//Final-Project
//image of dog
var imgD;
//image of fish
var imgF;
//for iterating through dog and fish photos
var num = 0;
//images of dog
var loadedImagesD = [];
//images of fish
var loadedImagesF = [];
//for dragging mouse
var xarray = [];
var yarray = [];
//variables for moth
var x;
var y;
var dx;
var dy;
//variables for blue moth
var w;
var z;
var dw;
var dz;
//variables for bee
var b;
var p;
var db;
var dp;
function preload() {
var backgroundImageURL = "https://i.imgur.com/qDrD310.png";
backgroundIMG = loadImage(backgroundImageURL);
var sunImageURL = "https://i.imgur.com/6SDAJjt.png";
sunIMG = loadImage(sunImageURL);
var mothImageURL = "https://i.imgur.com/bGUODEv.png?1";
mothIMG = loadImage(mothImageURL);
var bluemothImageURL = "https://i.imgur.com/FR3gCsQ.png";
bluemothIMG = loadImage(bluemothImageURL);
var beeImageURL = "https://i.imgur.com/oTcCy45.png";
beeIMG = loadImage(beeImageURL);
//dog photos
for (var i = 0; i < dogPhotos.length; i++) {
loadedImagesD.push(loadImage(dogPhotos[i]));
}
//fish photos
for (var i = 0; i < fishPhotos.length; i++) {
loadedImagesF.push(loadImage(fishPhotos[i]));
}
}
function setup() {
createCanvas(700, 500);
var d = floor(random(0, dogPhotos.length));
var f = floor(random(0, 0, fishPhotos.length));
//images of dog
imgD = loadedImagesD[d];
//images of fish
imgF = loadedImagesF[f];
x = random(width);
y = random(height);
dx = random(-5, 5);
dy = random(-5, 5);
w = random(width);
z = random(height);
dw = random(-5, 5);
dz = random(-5, 5);
b = random(width);
p = random(height);
db = random(-5, 5);
dp = random(-5, 5);
}
function draw() {
background(200);
//photo of background
image(backgroundIMG, 0, 0, 0);
//photo of sun
image(sunIMG, 0, 0, 0);
//photo of dog
image(imgD, 0, 0, imgD.width, imgD.height);
//photo of fish
image(imgF, 0, 0, imgF.width, imgF.height);
//drags smaller dog image along canvas
for (var i = 0; i < xarray.length; i++) {
fill(0);
image(imgD, xarray[i], yarray[i], imgD.width/2.4, imgD.height/2.4);
}
if (xarray.length > 8) {
xarray.shift(1);
yarray.shift(1);
}
//photo of moth
image(mothIMG, x, y, mothIMG.width/2.3, mothIMG.height/2.3);
x += dx;
y += dy;
//if image exceeds canvas width,
//set back to 0
if (x > width) x = 0;
else if (x < 0) x = width;
//if image exceeds canvas height,
//set back to 0
if (y > height) y = 0;
else if (y < 0) y = height;
//photo of blue moth
image(bluemothIMG, w, z, bluemothIMG.width/1.9, bluemothIMG.height/1.9);
w += dw;
z += dz;
//if image exceeds canvas width,
//set back to 0
if (w > width) w = 0;
else if (w < 0) w = width;
//if image exceeds canvas height,
//set back to 0
if (z > height) z = 0;
else if (z < 0) z = height;
//photo of bee
image(beeIMG, b, p, beeIMG.width/2.5, beeIMG.height/2.5);
b += db;
p += dp;
//if image exceeds canvas width,
//set back to 0
if (b > width) b = 0;
else if (b < 0) b = width;
//if image exceeds canvas height,
//set back to 0
if (p > height) p = 0;
else if (p < 0) p = height;
//lightens screen when mouse over sun
if (mouseX <= 398 & mouseX >= 320 && mouseY >= 30 && mouseY <= 105) {
rectMode(CORNER);
noStroke();
fill(246, 215, 161, 70);
rect(0, 0, 700, 500);
}
}
function mouseDragged() {
xarray.push(mouseX);
yarray.push(mouseY);
}
function mousePressed() {
//iterates through dog photos when mouse clicks its body
if (mouseX <= 500 & mouseX >= 150 && mouseY >= 275 && mouseY <= 400) {
var d = num % dogPhotos.length;
imgD = loadedImagesD[d];
num++;
}
//iterates through fish photos when mouse clicks blanket
if (mouseX <= 700 & mouseX >= 0 && mouseY >= 130 && mouseY <= 250) {
var f = num % fishPhotos.length;
imgF = loadedImagesF[f];
num++;
}
}
var dogPhotos = [
//rusty-colored dog
"https://i.imgur.com/2vglMnn.png",
//white/pink dog
"https://i.imgur.com/IWbYamo.png",
//lilac dog
"https://i.imgur.com/I5xU7LF.png",
//black dog
"https://i.imgur.com/AAQQgCj.png",
//blue dog
"https://i.imgur.com/CTZSr9D.png",
//mulberry dog
"https://i.imgur.com/kt5rN0R.png"]
var fishPhotos = [
//first fish
"https://i.imgur.com/bD3GBjE.png",
//second fish
"https://i.imgur.com/eUWa3dx.png",
//third fish
"https://i.imgur.com/XJFlBTd.png"]
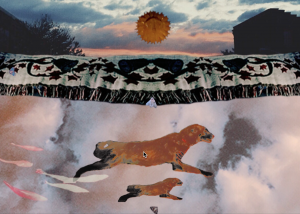
When I started this project, I knew that I wanted to give users a hands-on experience, similar to the I Spy computer games that I’d play as a kid, which I drew inspiration from. The worlds created in these games still felt genuine despite the fact that they were within a computer screen. This is in part because photos from real life were used.
Moreover, I really loved the Mixies Assignment and that it felt like we were playing with puzzle pieces and working with our hands, even though we were doing this electronically. This is why I chose to use photos that I’ve taken and use similar methods from that assignment to make this project interactive and fun.
To download this project, click this zip file: chickoff-final-project