sijings-wallpaper
var topSw1=14;//for house roof, x axis
var topSw2=5;//for house's left wall, x axis
var topSw3=23;//for house's right wall, x axis
var topSw=30;//for houseRoof's weight
var topSh1=0;//for house roof, y axis
var topSh2=5;//for house's left and right wall, y axis
var topSh=25;//for houseRoof's height
var numofRow1=15;//for defining numbers of loop
var numofCol1=20;
var locFx=14;//for flower's x axis
var locFy=20;//for flower's y axis
var locYc=30;//flower's height in between
var locXc=1.25;//flower's weight
var flowerW=11;//flower's petal's size
function setup() {
createCanvas(480, 455);
}
function draw() {
scale(1.3);
background(255);
var r1=230;
var b1=138;
var g1=92;
for (var row1=0; row1<numofRow1;row1++){
for (var col1=0; col1<numofCol1;col1++){
noStroke();
r1=r1+0.2;//for gradiant color
b1=b1+0.3;
g1=g1+0.3;
fill(r1,b1,g1);
if (row1%2!=0){//for setting the condition
topSw1=30;//shifting between rows constantly
}else{
topSw1=15;
}
var x1=topSw1+col1*topSw;
var y1=topSh1+row1*topSh-10;//based on calculation for eight points
var x2=topSw1+col1*topSw+15;
var y2=topSh1+row1*topSh;
var x3=topSw1+col1*topSw;
var y3=topSh1+row1*topSh+10;
var x4=topSw1+col1*topSw-15;
var y4=topSh1+row1*topSh;
quad(x1,y1,x2,y2,x3,y3,x4,y4)
}
}
var r2=225;//color variable for the walls
var b2=205;
var g2=203;
var r3=240;
var b3=240;
var g3=240;
for (var row2=0; row2<numofRow1;row2++){
for (var col2=0; col2<numofCol1;col2++){
noStroke();
r2=r2+0.2;//changing varaibles for the color
b2=b2+0.1;
g2=g2+0.1;
fill(r2,b2,g2);
if (row2%2!=0){//for changing positions
topSw2=20.5;
topSw3=39;
}else{
topSw2=5;
topSh2=5;
topSw3=23;
}
var x11=topSw2+col2*topSw-5;//for setting up the eight points
var y11=topSh2+row2*topSh-5;
var x21=topSw2+col2*topSw+10;
var y21=topSh2+row2*topSh+5;
var x31=topSw2+col2*topSw+10;
var y31=topSh2+row2*topSh+20;
var x41=topSw2+col2*topSw-5;
var y41=topSh2+row2*topSh+10;
quad(x11,y11,x21,y21,x31,y31,x41,y41);
r3=r3-0.2;//for the second side wall
b3=b3-0.1;
g3=g3-0.1;
fill(r3,b3,g3);
var x12=topSw3+col2*topSw-9;//for setting up the eight points
var y12=topSh2+row2*topSh+4;
var x22=topSw3+col2*topSw+7;
var y22=topSh2+row2*topSh-6;
var x32=topSw3+col2*topSw+11;
var y32=topSh2+row2*topSh+8;
var x42=topSw3+col2*topSw-8;
var y42=topSh2+row2*topSh+20;
if (col2==numofCol1-1){
x32=topSw3+col2*topSw+7;
y32=topSh2+row2*topSh+10;
}
quad(x12,y12,x22,y22,x32,y32,x42,y42);
}
}
var r4=187;
var g4=116;
var b4=52;
//green flower, flower roots, and door
for (var row3=0; row3<numofRow1;row3++){
for (var col3=0; col3<numofCol1;col3++){
noStroke();
fill(96,185,165);
if (row3%2!=0){
locFx=26;
}else{
locFx=10;
}
var e1=locFx+locYc*col3;
var e2=locFy*locXc*row3;
angleMode(DEGREES);//change radians to degree
arc(e1, e2, flowerW, flowerW, -50, 10, PIE);
arc(e1, e2, flowerW, flowerW, 35, 90, PIE);
arc(e1, e2, flowerW, flowerW, 110, 155, PIE);
arc(e1, e2, flowerW, flowerW, 175, 220, PIE);
strokeWeight(1);
stroke(87,148,83);
noFill();
curve(e1+30,e2+35,e1, e2, e1+7, e2, e1+15, e2+20);//the flower roots
noStroke();
//door and door handle
r4+=0.2;
g4+=0.2;
b4+=0.2;
fill(r4,g4,b4);
if (row3%2!=0){
topSw2=20.5;
topSw3=39;
}else{
topSw2=5;
topSh2=5;
topSw3=23;
}
var x1=topSw2+col3*topSw;
var y1=topSh2+row3*topSh+4;
var x2=topSw2+col3*topSw+5;
var y2=topSh2+row3*topSh+8;
var x3=topSw2+col3*topSw+5;
var y3=topSh2+row3*topSh+18;
var x4=topSw2+col3*topSw-1;
var y4=topSh2+row3*topSh+13;
quad(x1,y1,x2,y2,x3,y3,x4,y4);
fill(255);
ellipse(x1+3,y1+8,3,3);
}
}
}
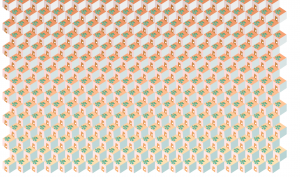
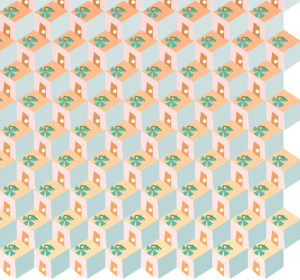
For this project, my inspiration came from the two-dimensional way of expressing three-dimensional object. The whole pattern is meant to be a set of houses with a plant living on top of it. The only problem I met was that I ignored the dimension I suppose to use for the project and used a much larger dimension. The actual pattern I created is larger than what I have now. Overall, I am happy about learning for loop, it makes my concept to another level.