amui1-p11
//Allison Mui
//15-104 Section A
//amui1@andrew.cmu.edu
//Project-11
//
//variables for rain
var x = 30;
var y = 30;
var xSpeed = 0.5;
var ySpeed = 3;
var treex = 10;
function setup() {
createCanvas(480,480);
}
function draw() {
background(51,79,102);
noStroke();
fill(178,198,204);
//make rows of rain
for (row = 0; row < 10; row++) {
ellipse(x + row*50,y,5,10);
//make columns of rain
for (col = 0; col < 5; col++) {
ellipse(x+row*50,y-80*col,5,10);
}
}
//move rain a different way based on location
if (y < height/4){
x -= xSpeed;
}
//move rain a different way based on location
if (y > height/4){
x += xSpeed;
}
//move rain down canvas
y += ySpeed;
//if rain off the page, go back to top
if (y > height/2+10) {
y = 0;
}
//if mouse is pressed, then show lightning
if (mouseIsPressed) {
lightning();
}
//move tree based on wind based on wind position
//reposition trunk and tree based on how wind is moving the tree
if (mouseX > width/2 - 10 & mouseX < width/2+10) {
wind = 118;
treew = 68;
trunkw = 90;
trunkDist = 50;
}
if (mouseX < width/2-10) {
wind = 110;
treew = 70;
trunkw = 80;
trunkDist = 50;
}
if (mouseX > width/2+10) {
wind = 135;
treew = 60;
trunkw = 100;
trunkDist = 38;
}
//make 5 trees
for (t = 0; t < 5; t++) {
turtleTree(treex+t*100,wind,treew,trunkw,trunkDist);
}
}
//make turtle for lightning
function lightning() {
light = makeTurtle(mouseX,mouseY);
light.setColor(color(233,255,103));
light.setWeight(5);
light.penDown();
light.forward(20);
light.right(115);
light.forward(30);
light.left(135);
light.forward(10);
light.right(140);
light.forward(30);
light.left(125);
light.forward(10);
light.right(130);
light.forward(40);
light.right(150);
light.forward(20);
light.left(115);
light.forward(10);
light.right(130);
light.forward(30);
light.left(120);
light.forward(10);
light.right(123);
light.forward(41);
light.penUp();
}
//make turtle tree
function turtleTree(treex,wind,treew,trunkw,trunkDist) {
trunk = makeTurtle(treex+25,height-trunkDist);
trunk.setColor(color(95,81,48));
trunk.setWeight(3);
trunk.penDown();
trunk.right(trunkw);
trunk.forward(48);
trunk.left(90);
trunk.forward(10);
trunk.left(90);
trunk.forward(48);
tree = makeTurtle(treex,height-50);
tree.setColor(color(93,134,67));
tree.setWeight(3);
tree.penDown();
tree.left(65);
tree.forward(15);
tree.left(125);
tree.forward(8);
tree.right(130);
tree.forward(15);
tree.left(125);
tree.forward(8);
tree.right(wind);
tree.forward(80);
tree.right(130);
tree.forward(80);
tree.left(230);
tree.forward(8);
tree.left(135);
tree.forward(15);
tree.left(240);
tree.forward(8);
tree.right(240);
tree.forward(20);
tree.right(122);
tree.forward(treew);
tree.penUp();
}
function turtleLeft(d){this.angle-=d;}function turtleRight(d){this.angle+=d;}
function turtleForward(p){var rad=radians(this.angle);var newx=this.x+cos(rad)*p;
var newy=this.y+sin(rad)*p;this.goto(newx,newy);}function turtleBack(p){
this.forward(-p);}function turtlePenDown(){this.penIsDown=true;}
function turtlePenUp(){this.penIsDown = false;}function turtleGoTo(x,y){
if(this.penIsDown){stroke(this.color);strokeWeight(this.weight);
line(this.x,this.y,x,y);}this.x = x;this.y = y;}function turtleDistTo(x,y){
return sqrt(sq(this.x-x)+sq(this.y-y));}function turtleAngleTo(x,y){
var absAngle=degrees(atan2(y-this.y,x-this.x));
var angle=((absAngle-this.angle)+360)%360.0;return angle;}
function turtleTurnToward(x,y,d){var angle = this.angleTo(x,y);if(angle< 180){
this.angle+=d;}else{this.angle-=d;}}function turtleSetColor(c){this.color=c;}
function turtleSetWeight(w){this.weight=w;}function turtleFace(angle){
this.angle = angle;}function makeTurtle(tx,ty){var turtle={x:tx,y:ty,
angle:0.0,penIsDown:true,color:color(128),weight:1,left:turtleLeft,
right:turtleRight,forward:turtleForward, back:turtleBack,penDown:turtlePenDown,
penUp:turtlePenUp,goto:turtleGoTo, angleto:turtleAngleTo,
turnToward:turtleTurnToward,distanceTo:turtleDistTo, angleTo:turtleAngleTo,
setColor:turtleSetColor, setWeight:turtleSetWeight,face:turtleFace};
return turtle;}
I had trouble coming up with a concept for this project. It was so open ended, that I wasn’t sure exactly what I should do. I managed to come to the idea of depicting a storm. The user can interact with the program by pressing the mouse to show lightning or move the mouse left and right to portray how wind would behave.
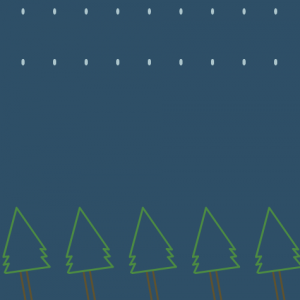
Caption: The user moved to the left side, as the “wind”, which swayed the trees on the bottom left.
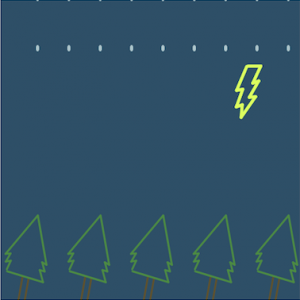
Caption: The user clicked the mouse and the lightning appeared.