sketch
var lamps = [];
var stars = [];
function setup() {
createCanvas(480, 280);
// create an initial collection of objects
for (var i = 0; i < 10; i++){
var rx = random(width);
lamps[i] = makeLamp(rx);
stars[i] = makeStar(rx);
}
frameRate(10);
}
function draw() {
background(37, 33, 90);
noStroke();
fill(209, 215, 41);
rect(0,height - 100,width,100);//ground
fill(195,206,60);
rect(0,height - 70, width, 70);
fill(181,200,52);
rect(0,height - 40, width, 40);
updateAndDisplayLamps();
removeLampsThatHaveSlippedOutOfView();
addNewLampsWithSomeRandomProbability();
updateAndDisplayStars();
removeStarsThatHaveSlippedOutOfView();
addNewStarsWithSomeRandomProbability();
for(var i = 190; i < height; i+=10){//draws lines in the foreground
stroke(181,200,52);
strokeWeight(1.5);
line(0, i, width, i);
}
}
function updateAndDisplayLamps(){
// Update the building's positions, and display them.
for (var i = 0; i < lamps.length; i++){
lamps[i].move();
lamps[i].display();
}
}
function updateAndDisplayStars(){
// Update the building's positions, and display them.
for (var i = 0; i < stars.length; i++){
stars[i].move();
stars[i].display();
}
}
function removeLampsThatHaveSlippedOutOfView(){
// takes away lamps once they leave the frame
var lampsToKeep = [];
for (var i = 0; i < lamps.length; i++){
if (lamps[i].x + lamps[i].breadth > 0) {
lampsToKeep.push(lamps[i]);
}
}
lamps = lampsToKeep; // remember the surviving buildings
}
function removeStarsThatHaveSlippedOutOfView(){
//removes stars from array stars and puts them in new array
//once they've moved off the edge of frame
var starsToKeep = [];
for (var i = 0; i < stars.length; i++){
if (stars[i].x + stars[i].breadth > 0) {
starsToKeep.push(stars[i]);
}
}
stars = starsToKeep; // remember the surviving stars
}
function addNewLampsWithSomeRandomProbability() {
// With a very tiny probability, add a new building to the end.
var newLampLikelihood = 0.013;
if (random(0,1) < newLampLikelihood) {
lamps.push(makeLamp(width));
}
}
function addNewStarsWithSomeRandomProbability() {
// With a very tiny probability, add a new building to the end.
var newStarLikelihood = 0.02;
if (random(0,1) < newStarLikelihood) {
stars.push(makeStar(width));
}
}
// method to update position of building every frame
function lampMove() {
this.x += this.speed;
}
function starMove() {
this.x += this.speed;
}
// draws lamps
function lampDisplay() {
fill(255,255,0,45);
noStroke()
; ellipse(this.x, height -100 - this.h,this.r,this.r); //halo of light from lamp
fill(102,166,218);
rect(this.x-1.5, height - 100 - this.h, this.breadth, this.h); //lamp post
fill(255);
ellipse(this.x, height - 100 - this.h, this.r2, this.r2); //lightbulb
stroke(255);
strokeWeight(0.5);
noFill();
quad(this.x - 1.5, height - 97 - this.h,
this.x - 5, height - 110 - this.h,
this.x + 5, height - 110 - this.h,
this.x + this.breadth - 1.5, height - 97 - this.h); //draws the lamp box
}
function starDisplay(){
//halo of light around star
noStroke();
fill(255, 255, 0, 30);
ellipse(this.x + this.breadth/2, this.h, this.breadth * 5, this.breadth * 5);
stroke(255,255,0);
strokeWeight(0.5);
noFill();
//draws diamonds that make up star
quad(this.x, this.h,
this.x + this.breadth / 2,this.h - this.tall / 2,
this.x + this.breadth, this.h,
this.x + this.breadth / 2,this.h + this.tall / 2);
//quad(this.x - this.breadth / 2, this.h,
//this.x + this.breadth, this.h + this.tall / 2,
//this.x + this.breadth / 2, this.h,
//this.x + this.breadth / 2, this.h - this.tall/2);
}
function makeLamp(posX) {
var lamp = {x: posX,
breadth: 3,
speed: -1.5,
h: random(40,60),
r: random(20,35),
r2: 4,
move: lampMove,
display: lampDisplay}
return lamp;
}
function makeStar(posX) {
var star = {x: posX,
breadth: 3,
speed: -0.5,
tall: random(5,10),
h: random(20, 100),
move: starMove,
display: starDisplay}
return star;
}
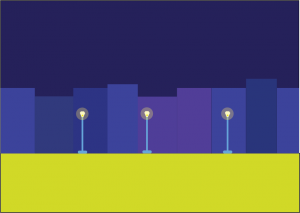
this was my original mock up in illustrator, but I struggled to get the building code to do what I wanted it to, so I decided to add other elements to supplement my lamps, so I added star objects, and made the foreground more detailed.