amui1-p6
//Allison Mui
//15-104 Section A
//amui1@andrew.cmu.edu
//Project-06
//variables for solar system
solarSize = 325;
//variables for star
function setup() {
createCanvas(400,400);
}
function draw() {
background(25,25,62);
//get time
var hr = hour();
var twelveHr = hr%12;
var min = minute();
var sec = second();
//print time
fill(255);
strokeWeight(0);
text(nf(twelveHr,2,0) + ':' + nf(min,2,0) + ':' + nf(sec,2,0),30,30);
//border of solar system
noFill();
stroke(60,60,85);
strokeWeight(6);
ellipse(width/2,height/2,solarSize,solarSize);
ellipse(width/2,height/2,solarSize-100,solarSize-100);
//HOURS
//flag stick
push();
translate(width/2,height/2);
rotate(hr);
stroke(255);
strokeWeight(5);
line(0,0,0,90);
pop();
//flag
push();
translate(width/2,height/2);
fill(255);
strokeWeight(0);
rotate(hr);
rectMode(CENTER);
fill(164,216,150);
rect(-25,78,40,30,5);
pop();
//flag symbol
push();
translate(width/2,height/2);
fill(255,255,0);
strokeWeight(0);
rotate(hr);
ellipse(-25,78,10,10);
pop();
//sun
//assign random variables to make sun move
var sunX = random(-.5,.5);
var sunY = random(-.5,.5);
fill(255,198,0);
strokeWeight(0);
ellipse(width/2,height/2,solarSize/4,solarSize/4);
//flares
for (i=0; i < 20; i++) {
push();
translate(width/2,height/2);
rotate(TWO_PI*i/20);
fill(255,172,0);
triangle(10+sunX,50+sunY,40-sunX,20+sunY,20+sunX,30+sunY);
pop();
}
//SECONDS
//star
fill(255,255,0);
//makes star move
var offset = second();
//maps second to width of the canvas
var offset = map(offset,0,60,0,width);
translate(0,50);
var xStar = [16+offset,20+offset,
30+offset,25+offset,
23+offset,15+offset,
7+offset,9+offset,
4+offset,14+offset];
var yStar = [5,10,
15,20,
30,25,
30,20,
15,10];
var lenStar = xStar.length;
beginShape();
for (var i = 0; i <lenStar; i++) {
vertex(xStar[i],yStar[i]);
}
endShape();
//shooting star
stroke(255);
strokeWeight(2);
line(-50+offset,15,0+offset,15);
line(-50+offset,20,0+offset,20);
//spaceship
fill(211,211,211);
strokeWeight(1);
//MINUTES
//makes spaceship move
var shipOff = minute();
var shipOff = map(shipOff,0,60,0,width);
//variables for ship x and y movement
var sxDist = 6;
var syDist = 10;
//to test
//print('subtracted=' + shipOff/syDist);
//draws ship
stroke(0);
translate(0,0);
//right side of circle
//left side of circle
//base triangle
triangle(-5+shipOff,height-90-shipOff/syDist,
27.5+shipOff,height-75-shipOff/syDist,
60+shipOff,height-90-shipOff/syDist);
//base little circles
ellipse(27.5+shipOff,height-73-shipOff/syDist,8,3);
ellipse(27.5+shipOff,height-70-shipOff/syDist,6,3);
ellipse(27.5+shipOff,height-66-shipOff/syDist,4,3);
//body of ship
strokeWeight(0);
//light pink
fill(249,170,202);
rect(-5+shipOff,height-95-shipOff/syDist,63,10,5);
//darker pink
fill(241,43,122);
rect(-10+shipOff,height-100-shipOff/syDist,75,8,5);
// top triangle
fill(172,153,198);
triangle(-5+shipOff,height-100-shipOff/syDist,
27.5+shipOff,height-120-shipOff/syDist,
60+shipOff,height-100-shipOff/syDist);
fill(209,209,227);
//top window thingy
arc(27.5+shipOff,height-106-shipOff/syDist,35,40,PI,0,CHORD);
//draws alien
//alien head
fill(164,216,150);
ellipse(27.5+shipOff,height-111-shipOff/syDist,10,10);
stroke(164,216,150);
strokeWeight(2);
//alien attenae
line(27.5+shipOff,height-111-shipOff/syDist,
23.5+shipOff,height-119-shipOff/syDist);
line(27.5+shipOff,height-111-shipOff/syDist,
31.5+shipOff,height-119-shipOff/syDist);
ellipse(22.5+shipOff,height-120-shipOff/syDist,5,1);
ellipse(33.5+shipOff,height-120-shipOff/syDist,5,1);
//alien eyes
fill(0);
strokeWeight(0);
ellipse(25.5+shipOff,height-112-shipOff/syDist,2,2);
ellipse(29.5+shipOff,height-112-shipOff/syDist,2,2);
}
I found this project to be one of the more challenging assignments. However, I liked it because there was a lot of room for creativity. Similar to the other projects, I wanted to tell time, but somehow include a story. I had the most trouble coordinating the rotations with the hours/seconds/minutes. In the future, I would like to spend more time expanding on that further.
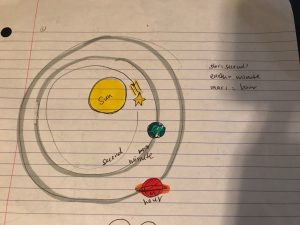
This was what my idea started out as.